Building a Flask Todo App: A Deep Dive into Architecture and Components
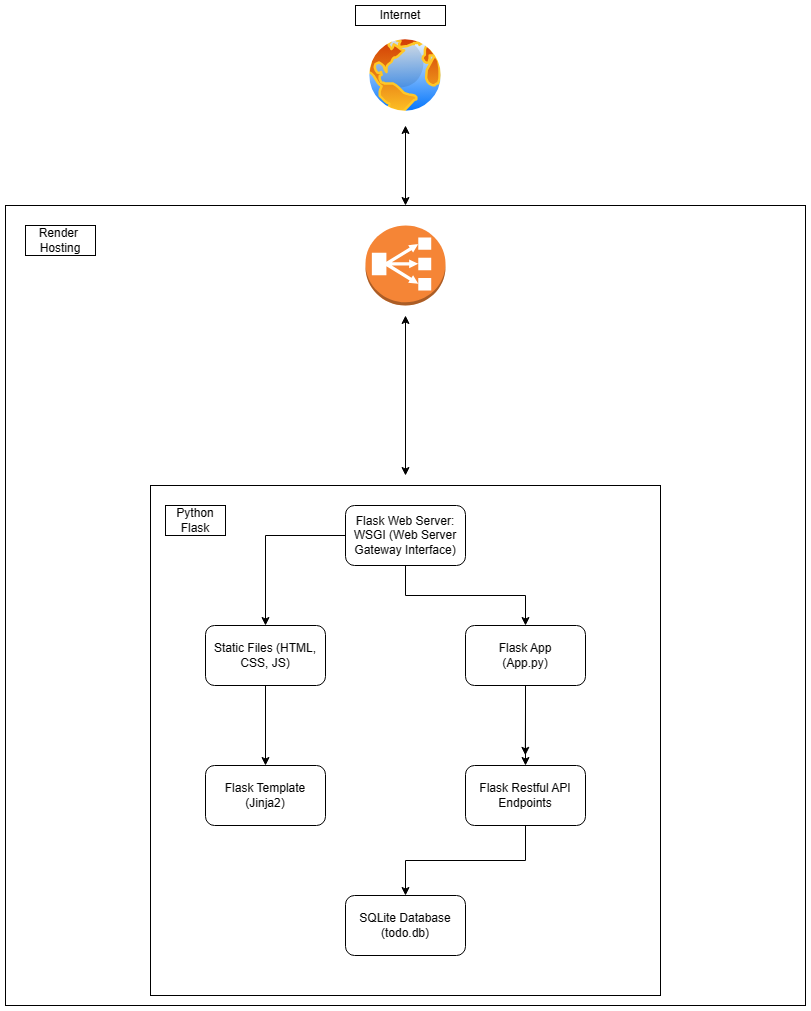
Introduction
In the realm of web development, Flask stands out as a lightweight and powerful framework for building web applications with Python. In this article, we'll delve into a practical example - a "Todo App" built using Flask. We'll explore the architecture, dissect the components, and understand how the various pieces come together to create a functional and scalable web application.
Project Overview
The Todo App is a simple yet effective task management system. Users can add, delete, and update tasks, providing a basic but essential example of web application functionality.
Project Structure
The project structure is divided into two main parts: the frontend, which encompasses the user interface (UI) presented in the web browser, and the backend, where the Flask application handles data management, storage, and business logic.
Frontend Components
User Interface (Web Browser)
The user interacts with the application through a web browser. The frontend is responsible for presenting the tasks, receiving user input, and displaying the results. Users access the web application via the Render hosting platform.
HTML, CSS, and JavaScript (Static Files)
Static files, including HTML, CSS, and JavaScript, are served to the user's browser by the web server. These files define the structure, style, and behavior of the Todo App's user interface.
Flask Templates (HTML)
Flask renders dynamic content using HTML templates. The templates, such as "index.html" for displaying tasks and "update.html" for updating tasks, provide a way to structure and present data to users.
Backend Components
Flask App (app.py)
The core of the Todo App is the Flask application, defined in the `app.py` script. Let's break down the key components and functionalities within this script.
Flask Initialization
from flask import Flask, render_template, url_for, request, redirect
from flask_sqlalchemy import SQLAlchemy
from datetime import datetime
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///todo_db.db'
db = SQLAlchemy(app)
Flask Initialization: The Flask app is created, and SQLAlchemy is configured to use an SQLite database named todo_db.db. SQLAlchemy is an Object-Relational Mapping (ORM) library that facilitates database interactions.
Todo Model
class Todo(db.Model):
id = db.Column(db.Integer, primary_key=True)
content = db.Column(db.String(200), nullable=False)
completed = db.Column(db.Integer, default=0)
data_created = db.Column(db.DateTime, default=datetime.utcnow)
def __repr__(self) -> str:
return '<Task %r>' % self.id
Todo Model: The Todo class represents the structure of the "Todo" table in the database. It includes fields like id, content, completed, and data_created.
Routes and Views
@app.route('/', methods=['POST', 'GET'])
def index():
# ... (Handling task creation and retrieval)
@app.route('/delete/')
def delete(id):
# ... (Handling task deletion)
@app.route('/update/', methods=['GET', 'POST'])
def update(id):
# ... (Handling task updating)
Routes and Views: The Flask app defines three routes - `/`, `/delete/
Database Operations
# ... (Database operations within route functions)
Database Operations: Within the route functions, database operations are performed using SQLAlchemy. Tasks are added, deleted, and updated within try-except blocks to catch and handle potential errors.
Running the App
if __name__ == '__main__':
app.run(debug=True)
Running the App: The app is configured to run in debug mode, which provides additional information for development purposes. In a production environment, this would be replaced with a more robust server setup.
Production Deployment Considerations
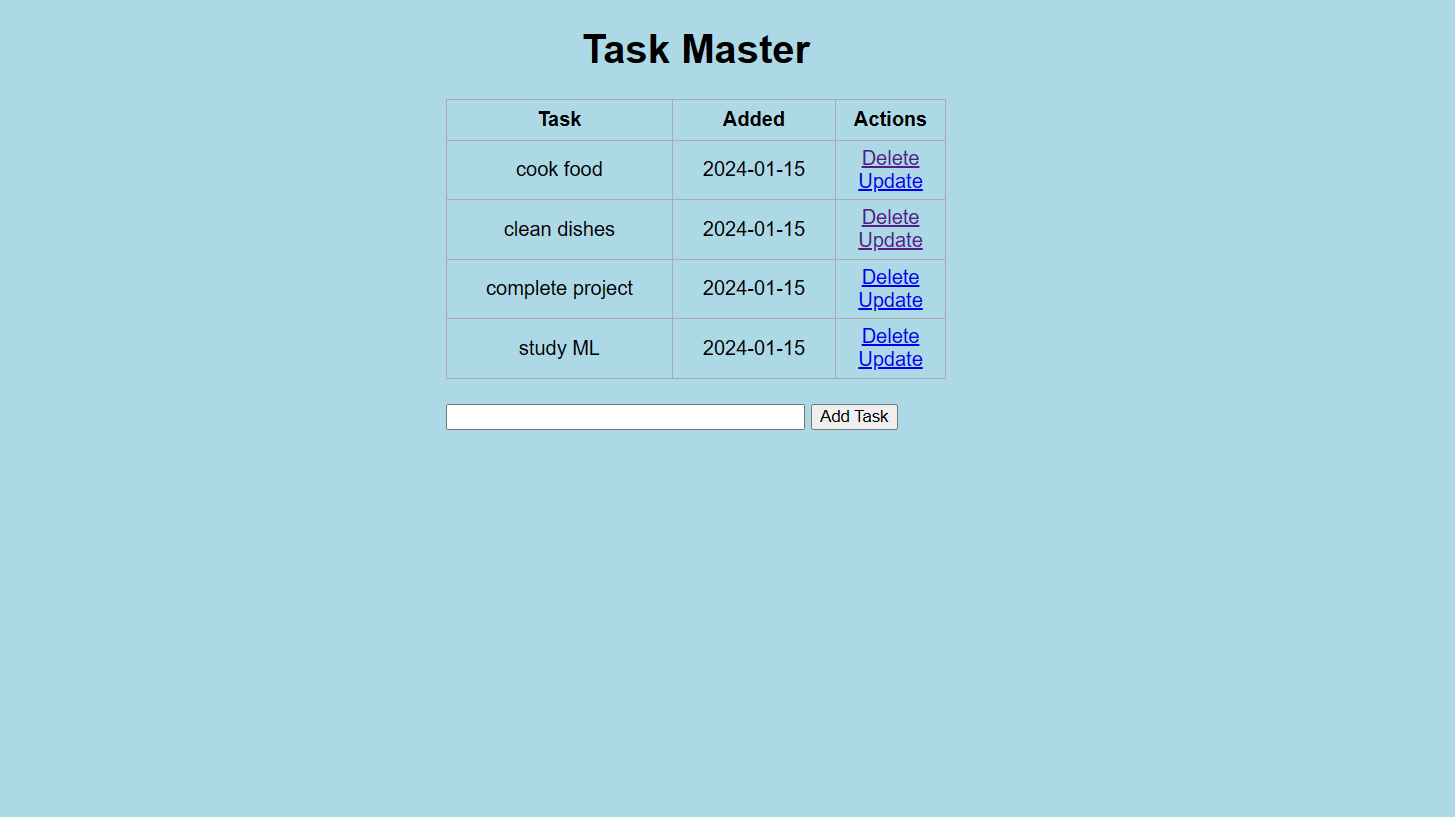
While the provided `app.py` is suitable for development, deploying it in a production environment requires additional considerations. In production, it's common to use a dedicated web server (e.g., Nginx or Apache) in conjunction with a WSGI server (e.g., Gunicorn or uWSGI).
Nginx/Apache as a Reverse Proxy
A production-ready web server, such as Nginx or Apache, acts as a reverse proxy. It forwards incoming HTTP requests to the WSGI server running the Flask application.
Gunicorn/uWSGI as the WSGI Server
A WSGI server handles the communication between the web server and the Flask application. Gunicorn and uWSGI are popular choices. For example, using Gunicorn:
gunicorn -w 4 -b 0.0.0.0:5000 app:app
This command runs the Flask app with 4 worker processes, binding to all available network interfaces.
Conclusion
In this deep dive, we've explored the architecture and components of a Flask Todo App. From the frontend user interface to the backend Flask application and database interactions, each piece plays a crucial role in creating a fully functional web application. Understanding these components is essential for developing robust and scalable web applications using Flask.
As you continue your journey in web development, consider enhancing the Todo App with additional features, security measures, and optimizations to further strengthen your skills in building real-world applications.
Please be aware that the website is hosted on a free tier, so it may take a few minutes (2-5 minutes) to load. Your patience is appreciated. Thank you for your understanding! 🕒🌐